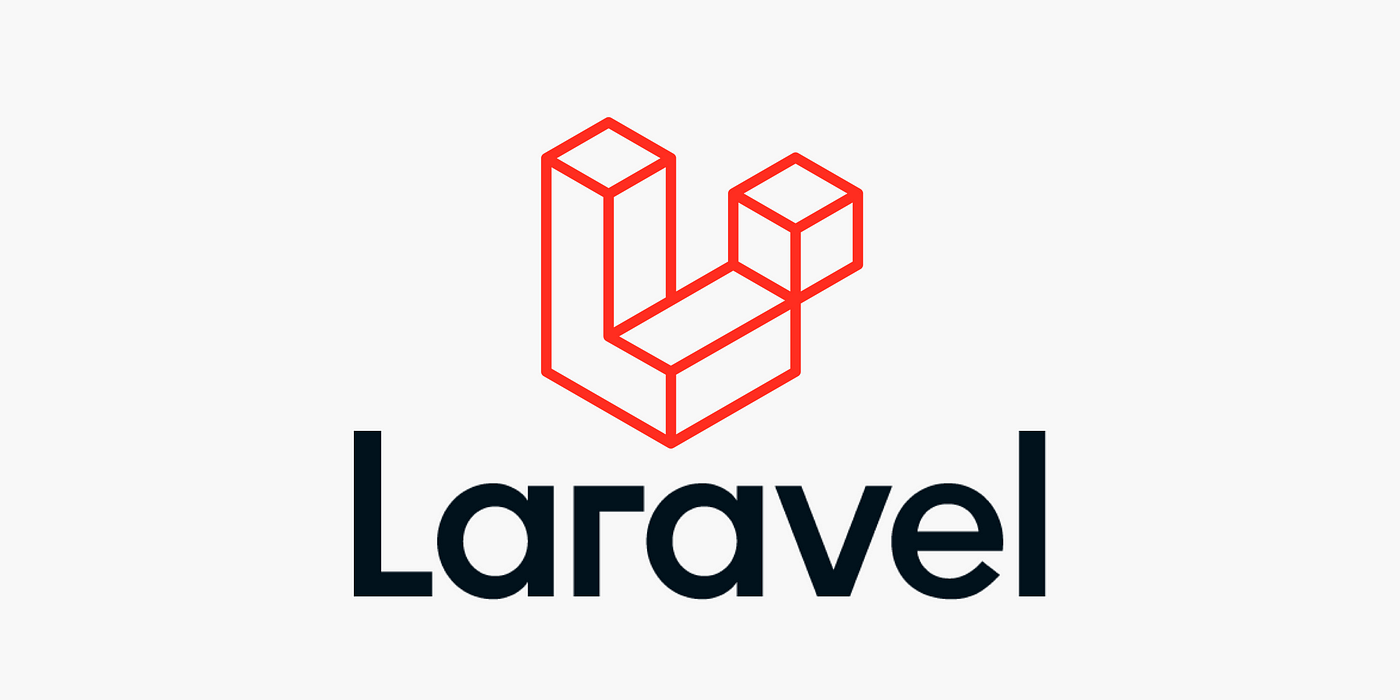
1. Make your code more elegant using Eloquent query scopes
$adminUsers = User::where('active', '=', 1)->where('is_admin', '=', 1)->get();
In order to make our code more readable and not be repetitive, we can use query scopes. For our example, we would create the following functions in our User model:
public function scopeActive(Builder $query): void
{
$query->where('active', true);
}
public function scopeAdmin(Builder $query): void
{
$query->where('admin', true);
}
Please visit https://laravel.com/docs/10.x/eloquent#query-scopes for further information.
2. Set column value automatically on model create
There are some situations in which you want to set a certain column automatically when creating a new record. In order to achieve this, you can use the model's creating event inside the boot method of the model.
The example below shows us creating a token when a newsletter entry is created.
class Newsletter extends Model
{
protected static function boot()
{
parent::boot();
Newsletter::creating(function(Newsletter $newsletter) {
$newsletter->token = Str::uuid()->toString();
});
}
}
The above will populate the token field on the newsletter when created with a UUID.
3. Avoid errors by using the optional helper
When accessing object values, if that object is null, your code will raise an error. For example:
return $invoice->total;
Would raise an error if the invoice object was empty. A simple way of avoiding errors is to use the optional Laravel helper:
return optional($invoice)->total;
Now, if the $invoice object is null, your code will return null instead of raising an error. You may also use a closure with the optional helper. It receives the closure as it's second argument and it will be called if the first argument is not null.
return optional(Invoice::find($id), function ($invoice) {
return $invoice->total;
});
Join my newsletter
Stay up to date with the latest trends, blogs and portfolio projects.